Attention
This website is still under construction.
Enigma 002 : The Four Hair Colours#
Are you ready to shift into second gear? At a carnival, Alice, Bob, Charlie, and Dahlia decide to enter a contest to win an inter galactic trip. The challenge? Each person must guess their own hair color. The team will have to work together to find the right strategy to determine the orange or indigo color of their hair. You will apply a new concept, the principle of parity.
Make sure to watch the following video before getting started with this exercise:
Important
On this website, you will be able to write and run your own Python code. To do so, you will need to click on the “Activate” button to enable all the code editors and establish a connection to a Kernel. Once clicked, you will see that the Status widget will start to show the connection progress, as well as the connection information. You are ready to write and run your code once you see Status:Kernel Connected
and kernel thebe.ipynb status changed to ready[idle]
just below. Please note that that refreshing the page in any way will cause you to lose all the code that you wrote. If you run into any issues, please try to reconnect by clicking on the “Activate” button again or reloading the page.
Run the cell below to install the necessary packages.
import sys !{sys.executable} -m pip install qiskit==1.1.1 !{sys.executable} -m pip install qiskit_aer==0.14.2 !{sys.executable} -m pip install pylatexenc==2.10 # Import necessary modules import numpy as np from qiskit import QuantumCircuit
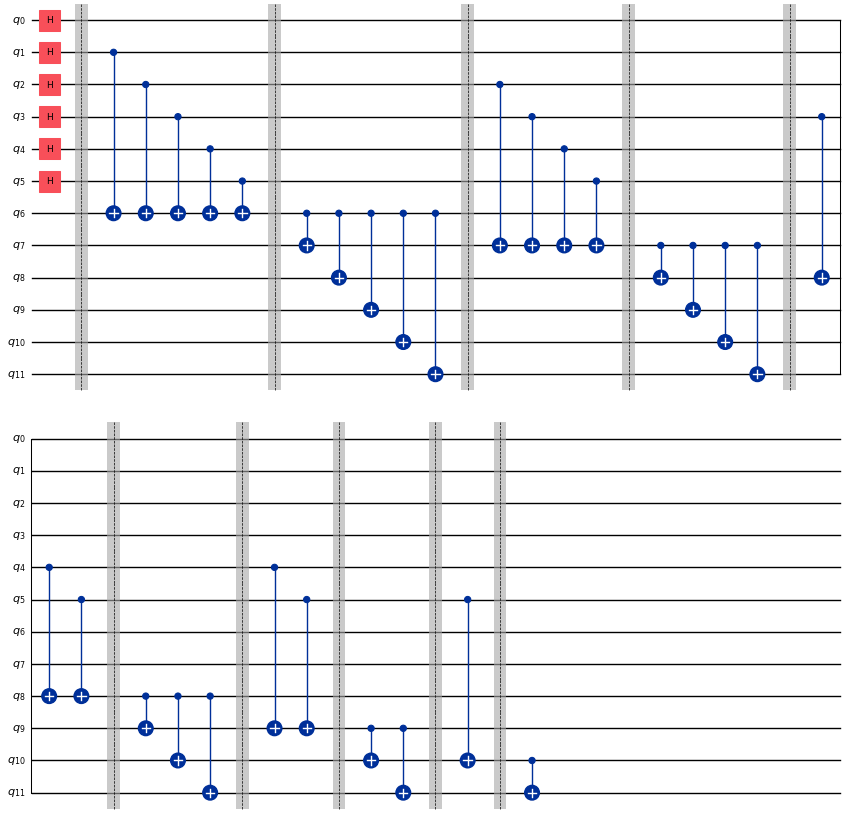
Exercise 1 - Code writing#
The enigma video presented a quantum circuit to solve the hair color problem with 4 people. Here’s an example of the code associated with the circuit:
problem_qc = QuantumCircuit(8)
problem_qc.h(0)
problem_qc.h(1)
problem_qc.h(2)
problem_qc.h(3)
problem_qc.barrier(0, 1, 2, 3, 4, 5, 6, 7)
# You check if the number of indigo hair color in front of you is even or odd
problem_qc.cx(1,4)
problem_qc.cx(2,4)
problem_qc.cx(3,4)
problem_qc.barrier(0, 1, 2, 3, 4, 5, 6, 7)
# Everyone takes note of the answer
problem_qc.cx(4,5)
problem_qc.cx(4,6)
problem_qc.cx(4,7)
problem_qc.barrier(0, 1, 2, 3, 4, 5, 6, 7)
# Bob checks the parity of the hair color in front of him
problem_qc.cx(2,5)
problem_qc.cx(3,5)
problem_qc.barrier(0, 1, 2, 3, 4, 5, 6, 7)
# Charlie and Dahlia take note of the answer
problem_qc.cx(5,6)
problem_qc.cx(5,7)
problem_qc.barrier(0, 1, 2, 3, 4, 5, 6, 7)
# Charkie checks the parity of Dahlia's hair color
problem_qc.cx(3,6)
problem_qc.barrier(0, 1, 2, 3, 4, 5, 6, 7)
# Dahlia takes note of Charlie's hair color
problem_qc.cx(6,7)
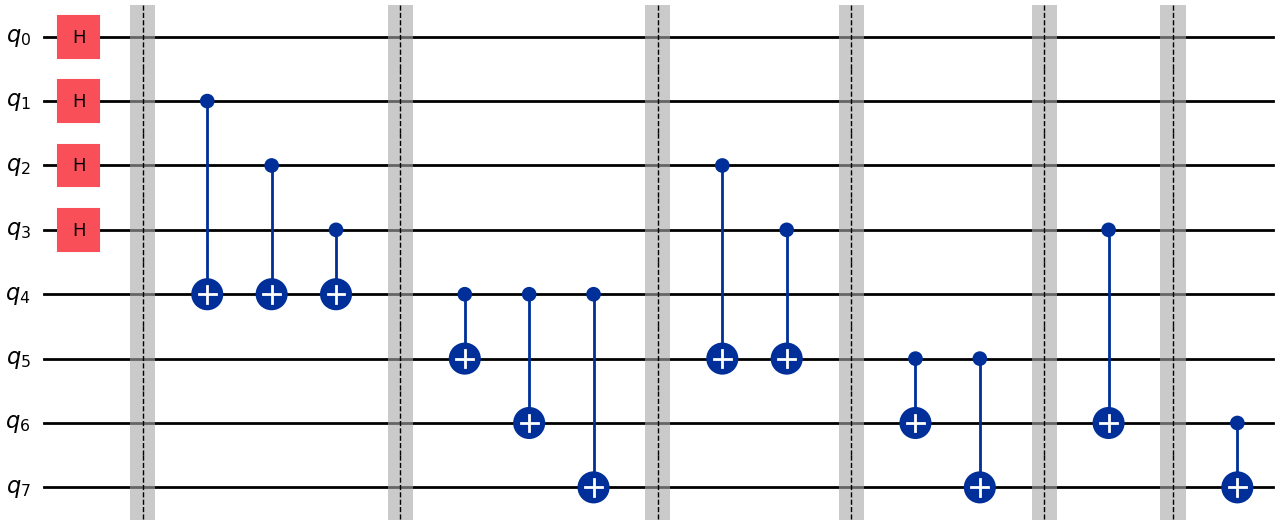
Can you adapt the circuit for 6 people?
problem_qc = QuantumCircuit(12) ### Start writing your code here. ### # Visualize the circuit problem_qc.draw('mpl')
Click to reveal the answer
problem_qc = QuantumCircuit(12)
problem_qc.h(0)
problem_qc.h(1)
problem_qc.h(2)
problem_qc.h(3)
problem_qc.h(4)
problem_qc.h(5)
problem_qc.barrier(0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11)
# You check if the number of indigo hair color in front of you is even or not
problem_qc.cx(1,6)
problem_qc.cx(2,6)
problem_qc.cx(3,6)
problem_qc.cx(4,6)
problem_qc.cx(5,6)
problem_qc.barrier(0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11)
# Everyone takes note of the answer
problem_qc.cx(6,7)
problem_qc.cx(6,8)
problem_qc.cx(6,9)
problem_qc.cx(6,10)
problem_qc.cx(6,11)
problem_qc.barrier(0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11)
# Bob checks the parity of the hair color in front of him
problem_qc.cx(2,7)
problem_qc.cx(3,7)
problem_qc.cx(4,7)
problem_qc.cx(5,7)
problem_qc.barrier(0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11)
# Everyone takes note of the answer
problem_qc.cx(7,8)
problem_qc.cx(7,9)
problem_qc.cx(7,10)
problem_qc.cx(7,11)
problem_qc.barrier(0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11)
# Charlie checks the parity of the hair color in front of him
problem_qc.cx(3,8)
problem_qc.cx(4,8)
problem_qc.cx(5,8)
problem_qc.barrier(0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11)
# Everyone takes note of the answer
problem_qc.cx(8,9)
problem_qc.cx(8,10)
problem_qc.cx(8,11)
problem_qc.barrier(0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11)
# Dahlia checks the parity of the hair color in front of her
problem_qc.cx(4,9)
problem_qc.cx(5,9)
problem_qc.barrier(0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11)
# Everyone takes note of the answer
problem_qc.cx(9,10)
problem_qc.cx(9,11)
problem_qc.barrier(0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11)
# Player E checks the parity of Player F hair's color
problem_qc.cx(5,10)
problem_qc.barrier(0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11)
# The last player finds his/her hair color depending on all the other players
problem_qc.cx(10,11)
# Visualize the circuit
problem_qc.draw('mpl')
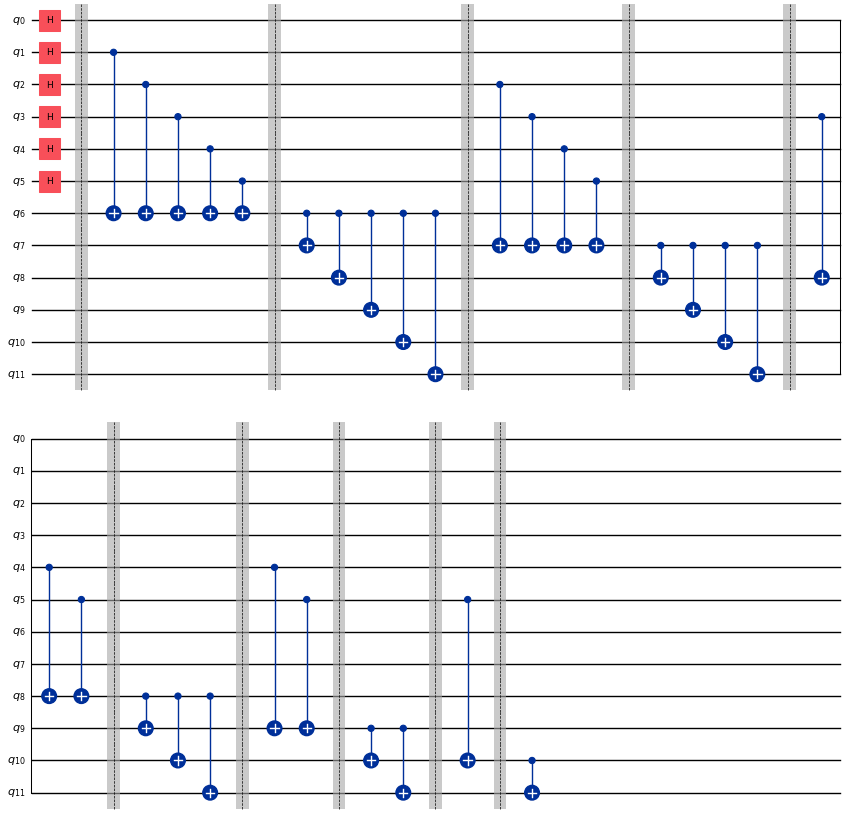
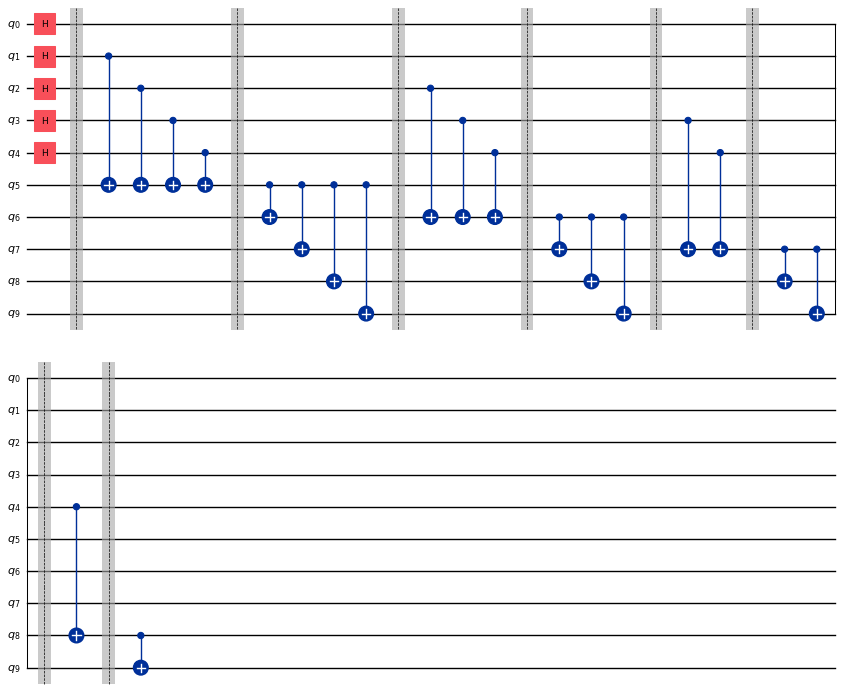
Exercise 2 - Code writing#
Simplify the code with a for
loop. Can you write a circuit for any number of people using a for loop?
nb_players = 5 nb_qubits = nb_players*2 problem_qc = QuantumCircuit(nb_qubits) for i in range(nb_players): problem_qc.h(i) start_qubit = 1 ### Add the rest of the code here. ### # Visualize the circuit problem_qc.draw('mpl')
Click to reveal the answer
nb_players = 5
nb_qubits = nb_players*2
problem_qc = QuantumCircuit(nb_qubits)
for i in range(nb_players):
problem_qc.h(i)
start_qubit = 1
for j in range(nb_players, nb_qubits-start_qubit):
problem_qc.barrier()
for i in range(start_qubit, nb_players):
problem_qc.cx(i, j)
problem_qc.barrier()
for k in range(j+1, nb_qubits):
problem_qc.cx(j, k)
start_qubit = start_qubit+1
# Visualize the circuit
problem_qc.draw('mpl')
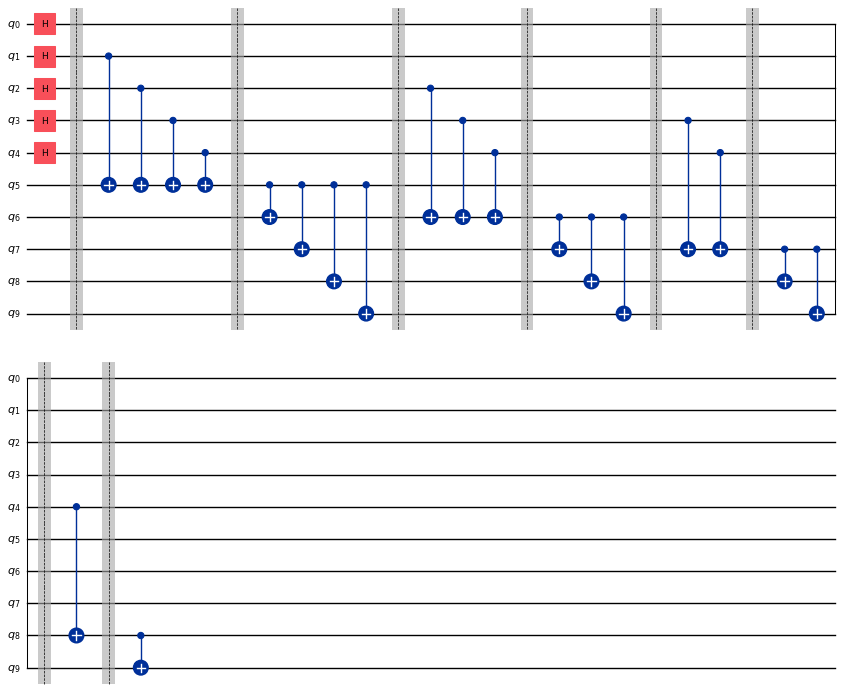
Exercise 3 - Quick quiz#
The goal of this enigma is to determine all the hair colors with the highest probability. Thus, what is the condition for 100% of the players to correctly guess their hair color?
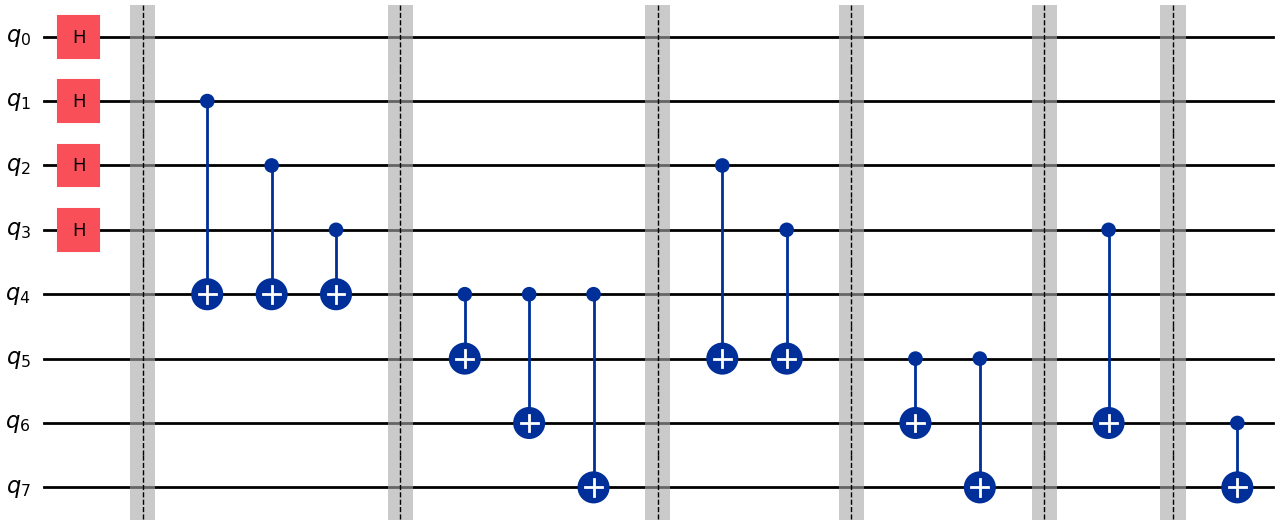
Exercise 4 - Quick quiz#
In all of the quantum circuits for this enigma, one qubit is never entangled. Which one is it?
Exercise 5 - Quick quiz#
Run the following code to execute the quantum circuit for 4 players on a simulator.
Note
When running quantum algorithms, simulators are often used to test the quantum circuits without monopolizing the ressources of a real quantum computer. Simulators are classical computers that mimic the behaviors of quantum computers.
# Quantum circuit for 4 players nb_players = 4 nb_qubits = nb_players*2 problem_qc = QuantumCircuit(nb_qubits) for i in range(nb_players): problem_qc.h(i) start_qubit = 1 for j in range(nb_players, nb_qubits-start_qubit): problem_qc.barrier() for i in range(start_qubit, nb_players): problem_qc.cx(i, j) problem_qc.barrier() for k in range(j+1, nb_qubits): problem_qc.cx(j, k) start_qubit = start_qubit+1 # Execute the circuit and draw the histogram measured_qc = problem_qc.measure_all(inplace=False) simulator = AerSimulator() result = simulator.run(transpile(measured_qc, simulator), shots=1024).result() counts = result.get_counts(measured_qc) plot_histogram(counts)